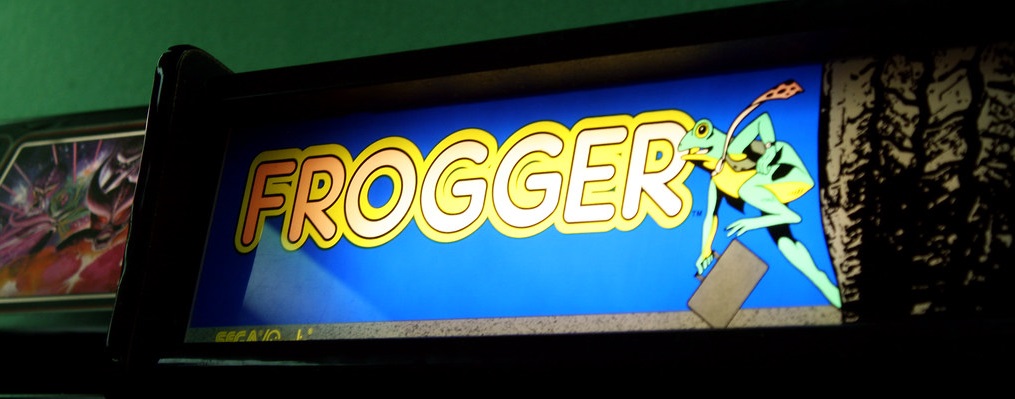
Game is an interactive way of using leisure and even passing time. People love to play games irrespective of age. When doing web development, why not build your own game to interact with your computer. When building a project like this you do not require higher knowledge of web development, but a good hold on your basics and something more in javascript.
We require three files namely index.html, style.css, app.js as the HTML, CSS, JavaScript files and that is enough. We are not focusing on the UI/UX that much it is the game we will build to learn and progress. A simple, yet time taking project where you may need to amend each file in sync with each other and not one by one.
Read more..
Looking to build projects on Game Development?:
Game Development Kit will be shipped to you and you can learn and build using tutorials. You can start for free today!
OUTLINE:
Frogger is a grid-based game, where the user is the frog that starts from the bottom of the grid and has to cross two obstacles to reach the ending block/ grid opposite side. The first obstacle is the road where cars move in both directions and we have to move avoiding being hit by any of them. Then is the second obstacle which is a river where to cross we have to hop on logs, just opposite of the previous one. This game is one of the few games seen in the non–multimedia mobiles.
THINGS WILL BE USED:
These are methods used in our javascript file:-
- querySelector()
- addEventListener()
- setInterval()
- clearInterval()
- forEach()
- classList.add()
- classList.contains()
- classList.remove()
REQUIREMENTS:
Latest projects on Game Development
Want to develop practical skills on Game Development? Checkout our latest projects and start learning for free
Hardware:
- One Laptop or Desktop with any OS (Linux/Windows/iOS)
Software/Technology:
- A Browser ( Chrome/Firefox/Opera/Safari/IE) to run the program. I prefer chrome or firefox as it provides a console to see behind the work of program like like the IDE of python ( to use just right-click on mouse and select ‘Inspect’ or Ctrl+Shift+I to open it and switch to console tag in this window)
- A text editor ( Visual Studio(VS) Code/Atom/Sublime/Notepad ) to code. I prefer VS code as it completes the code from its libraries and themes are attractive.
- Internet Connection
IMPLEMENTATION:
We will need three as mentioned before: i) index.html ii) style.css iii) app.js
We will be working simultaneously with each file in steps
STEP 1 ( building framework ):
- We start with setting up the HTML page. Adding title as ‘Frogger’, then linking CSS file using link tag and then linking javascript using a script tag
- we add our grid in div tag as a class in it, now add 81 div tags in between to make our grid
- add Start/Stop button with button class, Seconds left with span tag having class time-left for the timer, then we add h3 tag with the result as class
- Now we make the CSS part for this, to the grid we display as flex and flex-wrap as a wrap , the dimensions will be divisible by 9 like 180px*180px to get 9by9 grid
- for each division we give dimensions as per division by 9 like for above we give 20px*20px
- Then we give colour to the end block, start block and frog in grid
- now we hardcode these classes of end block and start block in div tag 5th down and above from grid div start and end respectively
STEP 2 ( forming the working ):
- now we add functionality to our frog in our javascript file using document addEventListener method where 'DOMContentLoaded', all our code will be done in this event listener
- we add all divs under variable squares using query selector All method to select ‘.grid div’ to select all divisions
- similarly we add other variables from html time left, start button, result
- we let current point at 76 as it is staring block point and declare width =9, also add current time to 20
- add ‘frog’ to current point using classList.add
- now we add function to move frog using the keyboard we will using key codes which can be found easily on google ( HINT: ‘37’: arrow left , ‘38’ : arrow up, ‘39’: arrow right, ‘40’: arrow down )
- see to move left decrease 1 and increase by 1 to move right in currentIndex
- while to to move down increase by width and decrease by width to move up
- now add frog at this index using the function
- Now going html page add following 19 from above same from below
from above
<div class="log-left l1"></div>
<div class="log-left l2"></div>
<div class="log-left l3"></div>
<div class="log-left l4"></div>
<div class="log-left l5"></div>
<div class="log-left l1"></div>
<div class="log-left l2"></div>
<div class="log-left l3"></div>
<div class="log-left l4"></div>
<div class="log-right l5"></div>
<div class="log-right l1"></div>
<div class="log-right l2"></div>
<div class="log-right l3"></div>
<div class="log-right l4"></div>
<div class="log-right l5"></div>
<div class="log-right l1"></div>
<div class="log-right l2"></div>
<div class="log-right l3"></div>
from below
<div class="car-right c1"></div>
<div class="car-right c2"></div>
<div class="car-right c3"></div>
<div class="car-right c1"></div>
<div class="car-right c2"></div>
<div class="car-right c3"></div>
<div class="car-right c1"></div>
<div class="car-right c2"></div>
<div class="car-right c3"></div>
<div class="car-left c1"></div>
<div class="car-left c2"></div>
<div class="car-left c3"></div>
<div class="car-left c1"></div>
<div class="car-left c2"></div>
<div class="car-left c3"></div>
<div class="car-left c1"></div>
<div class="car-left c2"></div>
<div class="car-left c3"></div>
- c1 is for cars c2,c3 for road and add in css
- l1,l2,l3 for logs and l4,l5 for river and color in css
Step 3 ( adding function of movement):
- declare constant variables for each type carleft , carright, logleft, logright and connect them with their classes using query selector All
- now make two auto functions one for autocar movement and other for aut log movement
- now we write functions to move cars go left for left and right for right , and in the same way for logs
- in each connect carleft variable with function to move car left and same for right using forEach method like
carsRight.forEach(carRight => moveCarRight(carRight));
- in same way call functions of log movement
- we have to declare four functions two for logs and two for cars movement
- In the carleft and carright we have three cases c1,c2,c3 checked using classList; changed using switch cases likewise c1->c2->c3->c1 for left and c1->c3,c2->c1,c3->c2 for right in following way
case carLeft.classList.contains('c1'):
carLeft.classList.remove('c1')
carLeft.classList.add('c2')
break
- In the same way we to do for logleft and logright but 5 cases , l1->l2->l3->l4->l5->l1 for left, l1->l5,l2->l1, l3->l2, l4->l3, l5->l4 for right
- now we set the rules to win and loose by declaring their functions
- in win function we check we if ending block contains frog get ‘YOU WIN’ as result on console using innerhtml
- now lose function has three condition if current time equals to zero or classList contains c1 the car or l4,l5 the river, we get output on console ‘You Lose’
- we clear the timer , remove frog, remove event using remove event listener
Step 4:
- we need two more functions for movement of a frog with the logs both left moving log and right moving logs
- it is simple we check the current index for frog if it is between log left logs indices (like in our case it was >=27 and <35) we increase the current index by 1 and for right log indices (like >18 and <26 ) we decrease current index by 1
- now we declare a function to make each function work each second called move pieces and add logic to decrease current time by 1 and update in the time left
- now using add event listener we use start/pause button to call move pieces to function with ‘click’ as the event
- we add an if-else statement to check the value of timeid, if equal to zero else update timer id as:-
if (timerId) {
clearInterval(timerId)
} else {
timerId = setInterval(movePieces, 1000)
document.addEventListener('keyup', moveFrog)
}
- change the frog CSS to last to show it
Now everything is done. It requires time, checking each step properly so that you render it well. And if you wish you can visit my Github for this and other projects
https://github.com/vaibhavkumar-779/
Did you know
Skyfi Labs helps students learn practical skills by building real-world projects.
You can enrol with friends and receive kits at your doorstep
You can learn from experts, build working projects, showcase skills to the world and grab the best jobs.
Get started today!
Kit required to develop Frogger game using JavaScript and HTML:
Technologies you will learn by working on Frogger game using JavaScript and HTML:
Frogger game using JavaScript and HTML
Skyfi Labs
•
Published:
2020-08-09 •
Last Updated:
2021-06-14