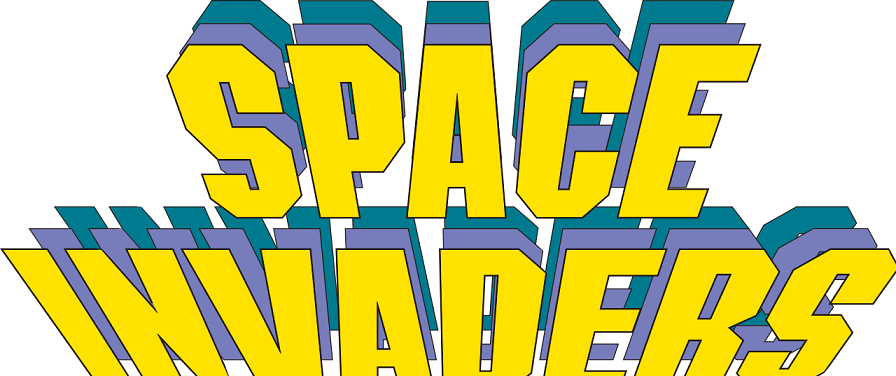
Game is an interactive way of using leisure and even passing time. People love to play games irrespective of age. When doing web development, why not build your own game to interact with your computer. When building a project thing like this you do not require higher knowledge of web development, but a good hold on your basics and something more in javascript.
We require three files namely index.html, style.css, app.js as the HTML, CSS, JavaScript files and that is enough. We are not focusing on the UI/UX that much it is the game we will build to learn and progress. A simple, yet time taking project where you may need to amend each file in sync with each other and not one by one.
Read more..
Looking to build projects on Game Development?:
Game Development Kit will be shipped to you and you can learn and build using tutorials. You can start for free today!
OUTLINE:
Space Invader is a simple game where the user as the shooter is expected to go to another side of the grid by destroying the three obstacles coming in between ( the grid would be 15x15 grid). This game is also like a time-bound game where there is no clock but the obstacles coming ahead become the counter of survival. This is largely based on Switch Cases. This game is one of the few games seen in the non–multimedia mobiles.
THINGS WILL BE USED:
These are methods used in our javascript file:-
- querySelector()
- addEventListener()
- Switch cases
- setInterval()
- clearInterval()
- keyCodes
- classList
- includes()
- push()
- indexOf()
REQUIREMENTS:
Hardware:
- One Laptop or Desktop with any OS (Linux/Windows/iOS)
Latest projects on Game Development
Want to develop practical skills on Game Development? Checkout our latest projects and start learning for free
Software/Technology:
- A Browser ( Chrome/Firefox/Opera/Safari/IE) to run the program. I prefer chrome or firefox as it provides a console to see behind the work of program like like the IDE of python ( to use just right-click on mouse and select ‘Inspect’ or Ctrl+Shift+I to open it and switch to console tag in this window)
- A text editor ( Visual Studio(VS) Code/Atom/Sublime/Notepad ) to code. I prefer VS code as it completes the code from its libraries and themes are attractive.
- Internet Connection
IMPLEMENTATION:
We will need three as mentioned before: i) index.html ii) style.css iii) app.js
We will be working simultaneously with each file in steps
Step 1:
- add basic structure of html and remember to link the css file (style.css) and javascript file (app.js)
- add h3 tag with name as ‘Score’ then span tag with id ‘result’
- now add div with class ‘grid’
- in that for 15x15 grid we add 225 div tags
- now in css to grid class add feature of grid as flex wrapping and dimensions as 300px X 300px
- for div under grid use dimension 20px X 20px
- in css add classes shooter (background: blue), invader (background:purple, radius 10px), boom (background:red), laser(background: orange)
Step2:
- now we add functionality to our frog in our javascript file using document addEventListener method where 'DOMContentLoaded', all our code will be done in this event listener
- we add all divs under variable squares using query selector All method to select ‘.grid div’ to select all divisions
- similarly, we add result display variable from HTML connecting with ‘#class’ query selector
- we let current shooter point at 202 as it is staring block point and declare width =15, also add current invader point to 0, result=0, array of aliens taken down =[], direction = 1
- we define alien Invaders with an array of numbers from 0 to 39, this define places where the invaders would be and we easily see them by forEach
- //draw alien invaders
alienInvaders.forEach(invader => squares[currentInvaderIndex + invader].classList.add('invader'));
- we can control this by changing the values like 0 to 5 in array see in the HTML page
- the same thing for shooter use classList and add shooter as above inside for each
Step3:
- now we add movement to shooter through function with a calling variable as ‘e’ as event
- ‘e’ denotes events with a key code for the keyboard buttons ( easily available on google )
- before putting in switch method remove shooter from the present position
- now we change position as per the cases of key pressed
- if we press left arrow (‘<-’) move left with key code = 37 and for right (‘->’) move right with key code = 39
- to activate this function we use addEventListener with ‘keydown’ as event call the move shooter function
- now check-in browser and proceed
- now we will bring movement in invaders using time looping
- we will fix left edge and right edge with comparison to the width
- now using if else if statement we give direction ( -1 for left and 1 for right )
- make direction = width to change line
- in else if check for change for line change and again use if else to check if on left edge so change direction to 1 else to -1
- now using for loop we remove invader from current position add direction and then again add invaders to new positions
- in the same function, we check game over in ways
- first using if statement we check if both invader and shooter are present, then display ‘game over’ and clear interval
- second using for loop we check if any alien is last 4 squares of grid we declare game over in the following way:
if (alienInvaders[i] > (squares.length - (width - 1))) {
resultDisplay.textContent = 'Game Over'
clearInterval(invaderId)
}
- done for invader cross-check on browser
Step4:
- Now we work on shooting
- first we declare laserId, then currentLaserIndex equal currentShooterIndex
- now we add the function within this function to move laser
- we first remove laser from current laser position then decrease index value by width taking the line up and add laser here
- now we check if at current position we have any invader with laser, so we remove laser and invader both and add boom at this position
- now we set timeout to remove boom after 250 milliseconds like:
setTimeout(() => squares[currentLaserIndex].classList.remove('boom'), 250);
clearInterval(laserId);
- then we declare a variable for carrying of alientaken down and push it into array of alieninvadertakendown declared much before
- now we increase result by 1 and update resultdisplay to increase score and move out of if statement
- lastly we check if any of the laser have reached the last row or squares we remove laser from the index
- now out of the we declare the calling laser function using SPACEBAR (key code 32 )
document.addEventListener('keyup', e => {
if (e.keyCode) {
laserId = setInterval(moveLaser, 100);
}
})
- now we close the whole and set calling the function like this
document.addEventListener('keyup', shoot)
- we need to change the last for statement we used in last step while declaring the function moveInvaders (this is because we have an array to compare, otherwise the aliens will re emerge even after shooting them )
- from this below:
for (i = 0; i < alienInvaders.length - 1; i++) {
squares[alienInvaders[i]].classList.add('invader');
}
for (i = 0; i < alienInvaders.length - 1; i++) {
if (!alienInvadersTakenDown.includes(i)) {
squares[alienInvaders[i]].classList.add('invader');
}
}
- now in the function move invader we add win criteria ( logic = if all invaders killed we win)
//decide win
if (alienInvadersTakenDown.length === alienInvaders.length) {
resultDisplay.textContent = 'YOU WIN';
clearInterval(invaderId);
}
Now we are done with coding, you can change shape, size, and way of movement, add some looping, increase the grid, remember divisions calculations, improve upon it. You can refer to my Github profile for help:
https://github.com/vaibhavkumar-779/
Did you know
Skyfi Labs helps students learn practical skills by building real-world projects.
You can enrol with friends and receive kits at your doorstep
You can learn from experts, build working projects, showcase skills to the world and grab the best jobs.
Get started today!
Kit required to develop Space Invader game using HTML, CSS and JavaScript:
Technologies you will learn by working on Space Invader game using HTML, CSS and JavaScript:
Space Invader game using HTML, CSS and JavaScript
Skyfi Labs
•
Published:
2020-08-09 •
Last Updated:
2021-07-03